Framework
Theme
All
683
Forms
16
Charts
21
Navbars
45
Sidebars
27
Typography
42
Components
62
Tables
34
Cards
70
Contact Us
9
Testimonials
19
Features
25
Cards Section
38
Teams Section
11
Faq
9
Headers
47
Blogs
15
Contacts Section
9
Profiles Section
13
Charts Section
7
Tables Section
15
Contents
18
Logo Areas
12
Projects Section
14
Stats
13
Authentication
15
Call To Actions
21
Http Codes
6
Teams
16
Pricing
13
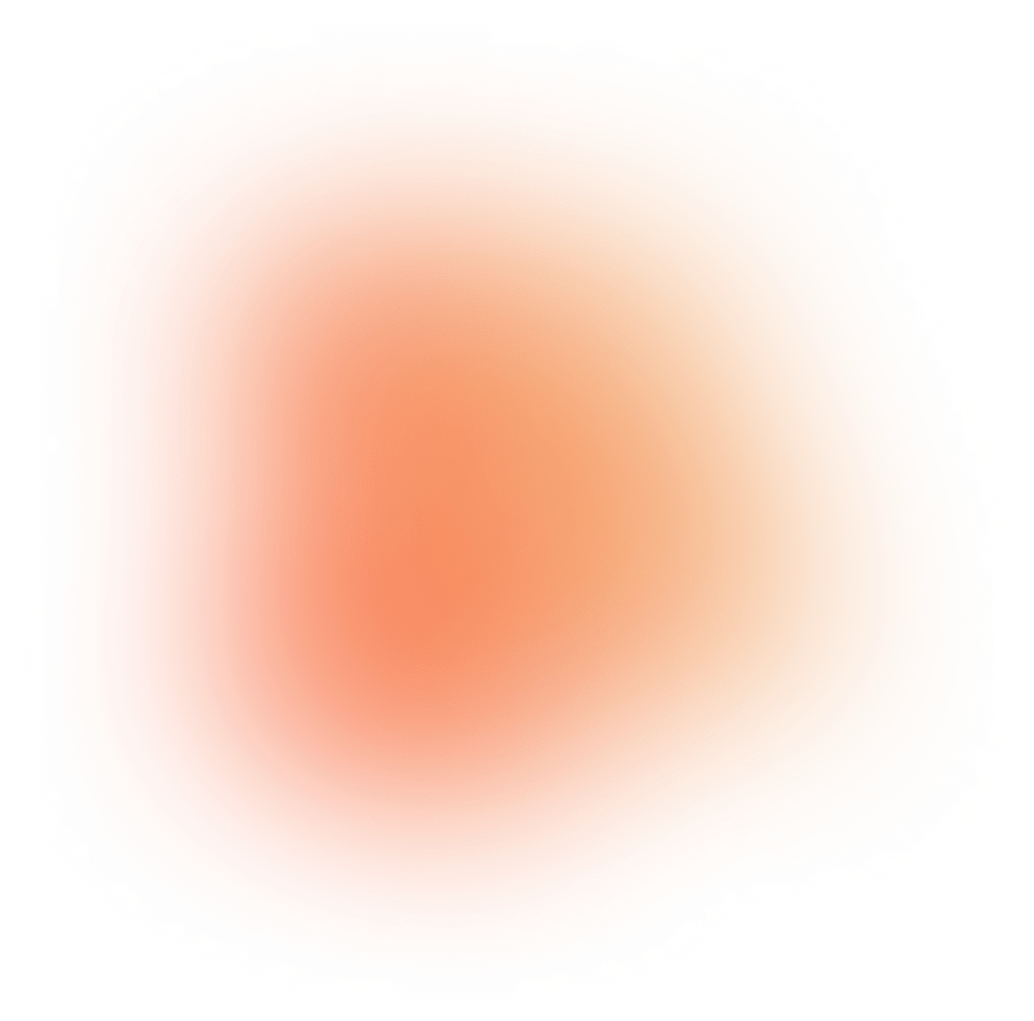
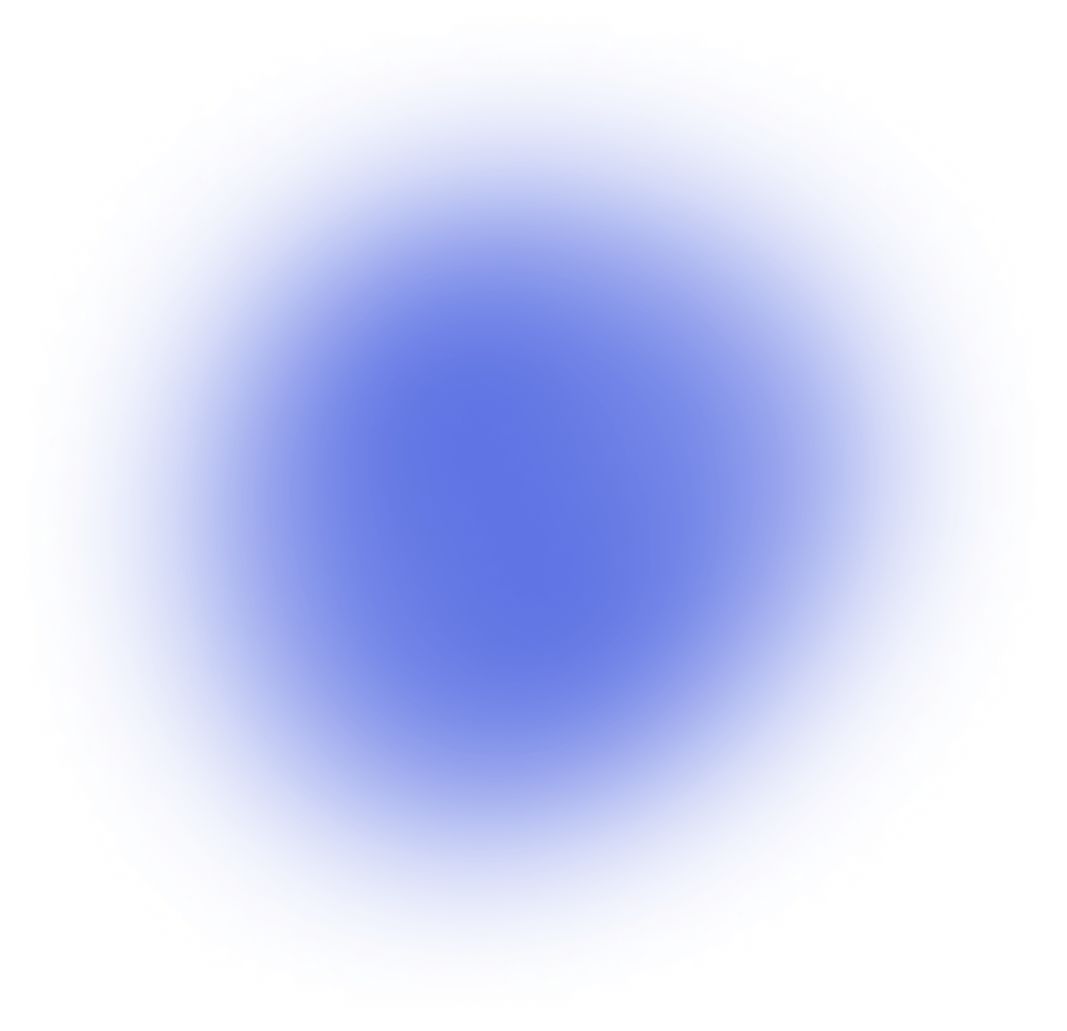
Charts - All Examples
Discover 21 Charts examples available in Loopple
Requires JavaScript
Chart Line
Component from
Soft UI Dashboard
Builder
<div class="card draggable"> <div class="card-header pb-0"> <h6>Sales overview</h6> <p class="text-sm"> <i class="fa fa-arrow-up text-success" aria-hidden="true"></i> <span class="font-weight-bold">4% more</span> in 2021 </p> </div> <div class="card-body p-3"> <div class="chart"><div class="chartjs-size-monitor"><div class="chartjs-size-monitor-expand"><div class=""></div></div><div class="chartjs-size-monitor-shrink"><div class=""></div></div></div> <canvas id="chart-line" class="chart-canvas chartjs-render-monitor chart-line" height="600" width="1088" style="display: block; height: 300px; width: 544px;"></canvas> </div> </div> </div>
<script>
if (document.querySelector(".chart-line")) { var chartsLine = document.querySelectorAll(".chart-line"); chartsLine.forEach(function(chart) { var ctx = chart.getContext("2d"); var gradientStroke1 = ctx.createLinearGradient(0, 230, 0, 50); gradientStroke1.addColorStop(1, "rgba(203,12,159,0.2)"); gradientStroke1.addColorStop(0.2, "rgba(72,72,176,0.0)"); gradientStroke1.addColorStop(0, "rgba(203,12,159,0)"); //purple colors var gradientStroke2 = ctx.createLinearGradient(0, 230, 0, 50); gradientStroke2.addColorStop(1, "rgba(20,23,39,0.2)"); gradientStroke2.addColorStop(0.2, "rgba(72,72,176,0.0)"); gradientStroke2.addColorStop(0, "rgba(20,23,39,0)"); //purple colors if (!chart.getAttribute('data-chart-initialized')) { new Chart(ctx, { type: "line", data: { labels: ["Apr", "May", "Jun", "Jul", "Aug", "Sep", "Oct", "Nov", "Dec"], datasets: [{ label: "Mobile apps", tension: 0.4, borderWidth: 0, pointRadius: 0, borderColor: "#cb0c9f", borderWidth: 3, backgroundColor: gradientStroke1, fill: true, data: [50, 40, 300, 220, 500, 250, 400, 230, 500], maxBarThickness: 6, }, { label: "Websites", tension: 0.4, borderWidth: 0, pointRadius: 0, borderColor: "#3A416F", borderWidth: 3, backgroundColor: gradientStroke2, fill: true, data: [30, 90, 40, 140, 290, 290, 340, 230, 400], maxBarThickness: 6, }, ], }, options: { responsive: true, maintainAspectRatio: false, plugins: { legend: { display: false, }, }, interaction: { intersect: false, mode: "index", }, scales: { y: { grid: { drawBorder: false, display: true, drawOnChartArea: true, drawTicks: false, borderDash: [5, 5], }, ticks: { display: true, padding: 10, color: "#b2b9bf", font: { size: 11, family: "Open Sans", style: "normal", lineHeight: 2, }, }, }, x: { grid: { drawBorder: false, display: false, drawOnChartArea: false, drawTicks: false, borderDash: [5, 5], }, ticks: { display: true, color: "#b2b9bf", padding: 20, font: { size: 11, family: "Open Sans", style: "normal", lineHeight: 2, }, }, }, }, }, }); chart.setAttribute("data-chart-initialized", "true"); } }); };
</script>
Requires JavaScript
Chart Bar
Component from
Argon Dashboard
Builder
<div class="card draggable"> <div class="card-header bg-transparent"> <h6 class="text-uppercase text-muted ls-1 mb-1">Performance</h6> <h5 class="h3 mb-0">Total orders</h5> </div> <div class="card-body"> <div class="chart"> <canvas id="chart-bars" class="chart-bar chart-canvas"></canvas> </div> </div> </div>
<script>
var chartsBar = document.querySelectorAll(".chart-bar"); chartsBar.forEach(function(chart) { if (!chart.getAttribute('data-chart-initialized')) { new Chart(chart, { type: "bar", data: { labels: ["Jul", "Aug", "Sep", "Oct", "Nov", "Dec"], datasets: [ { label: "Sales", tension: 0.4, borderWidth: 0, pointRadius: 0, backgroundColor: "#fb6340", data: [25, 20, 30, 22, 17, 29], maxBarThickness: 10, }, ], }, options: { responsive: true, maintainAspectRatio: false, legend: { display: false, }, tooltips: { enabled: true, mode: "index", intersect: false, }, scales: { yAxes: [ { gridLines: { borderDash: [2], borderDashOffset: [2], drawTicks: false, drawBorder: false, lineWidth: 1, zeroLineWidth: 0, zeroLineBorderDash: [0], zeroLineBorderDashOffset: [2], }, ticks: { beginAtZero: true, padding: 10, fontSize: 13, lineHeight: 5, fontColor: "#8898aa", fontFamily: "Open Sans", }, }, ], xAxes: [ { gridLines: { display: false, drawBorder: false, drawOnChartArea: false, drawTicks: false, }, ticks: { padding: 20, fontSize: 13, fontColor: "#8898aa", fontFamily: "Open Sans", }, }, ], }, }, }); chart.setAttribute("data-chart-initialized", "true"); } });
</script>
Requires JavaScript
Chart Bar Stacked
Component from
Asteria Dashboard
Builder
<div class="card mb-4 z-index-2 draggable"> <div class="card-header pb-0"> <h6 class="mb-1">Stock Available</h6> </div> <div class="card-body"> <canvas class="chart-bar-stacked" width="400" height="200"></canvas> </div> </div>
<script>
const ctx3 = document.querySelectorAll('.chart-bar-stacked'); const data = { labels: [ "2015", "2016", "2017", "2018", "2019", "2020" ], datasets: [ { label: "Long", backgroundColor: "#0dcaf0", data: [ 9000, 5000, 5240, 3520, 2510, 3652 ] }, { label: "Short", backgroundColor: "#5e72e4", data: [ 3000, 4000, 6000, 3500, 3600, 8060 ] }, { label: "Spreading", backgroundColor: "#20c997", data: [ 6000, 7200, 6500, 4600, 3600, 9200 ] } ] }; const options = { scales: { yAxes: [ { stacked: true, ticks: { fontSize: 14, lineHeight: 3, fontColor: "#adb5bd" }, gridLines: { display: false } }], xAxes: [ { stacked: true, ticks: { fontSize: 14, lineHeight: 3, fontColor: "#adb5bd" } } ] } }; const chart = new Chart(ctx3[ctx3.length-1], { // The type of chart we want to create type: "bar", // The data for our dataset data: data, // Configuration options go here options: options });
</script>
Requires JavaScript
Chart Bar
Component from
Soft UI Dashboard
Builder
<div class="card draggable"> <div class="card-body p-3"> <div class="bg-gradient-dark border-radius-lg py-3 pe-1 mb-3"> <div class="chart"><div class="chartjs-size-monitor"><div class="chartjs-size-monitor-expand"><div class=""></div></div><div class="chartjs-size-monitor-shrink"><div class=""></div></div></div> <canvas id="chart-bars" class="chart-bar chart-canvas chartjs-render-monitor" height="340" style="display: block; height: 170px; width: 368px;" width="736"></canvas> </div> </div> <h6 class="ms-2 mt-4 mb-0"> Active Users </h6> <p class="text-sm ms-2"> (<span class="font-weight-bolder">+23%</span>) than last week </p> <div class="container border-radius-lg"> <div class="row"> <div class="col-3 py-3 ps-0"> <div class="d-flex mb-2"> <div class="icon icon-shape icon-xxs shadow border-radius-sm bg-gradient-primary text-center me-2 d-flex align-items-center justify-content-center"> <svg width="10px" height="10px" viewBox="0 0 40 44" version="1.1" xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink"> <title>document</title> <g id="Basic-Elements" stroke="none" stroke-width="1" fill="none" fill-rule="evenodd"> <g id="Rounded-Icons" transform="translate(-1870.000000, -591.000000)" fill="#FFFFFF" fill-rule="nonzero"> <g id="Icons-with-opacity" transform="translate(1716.000000, 291.000000)"> <g id="document" transform="translate(154.000000, 300.000000)"> <path class="color-background" d="M40,40 L36.3636364,40 L36.3636364,3.63636364 L5.45454545,3.63636364 L5.45454545,0 L38.1818182,0 C39.1854545,0 40,0.814545455 40,1.81818182 L40,40 Z" id="Path" opacity="0.603585379"></path> <path class="color-background" d="M30.9090909,7.27272727 L1.81818182,7.27272727 C0.814545455,7.27272727 0,8.08727273 0,9.09090909 L0,41.8181818 C0,42.8218182 0.814545455,43.6363636 1.81818182,43.6363636 L30.9090909,43.6363636 C31.9127273,43.6363636 32.7272727,42.8218182 32.7272727,41.8181818 L32.7272727,9.09090909 C32.7272727,8.08727273 31.9127273,7.27272727 30.9090909,7.27272727 Z M18.1818182,34.5454545 L7.27272727,34.5454545 L7.27272727,30.9090909 L18.1818182,30.9090909 L18.1818182,34.5454545 Z M25.4545455,27.2727273 L7.27272727,27.2727273 L7.27272727,23.6363636 L25.4545455,23.6363636 L25.4545455,27.2727273 Z M25.4545455,20 L7.27272727,20 L7.27272727,16.3636364 L25.4545455,16.3636364 L25.4545455,20 Z" id="Shape"></path> </g> </g> </g> </g> </svg> </div> <p class="text-xs mt-1 mb-0 font-weight-bold">Users</p> </div> <h4 class="font-weight-bolder">36K</h4> <div class="progress w-75"> <div class="progress-bar bg-dark w-60" role="progressbar" aria-valuenow="60" aria-valuemin="0" aria-valuemax="100"></div> </div> </div> <div class="col-3 py-3 ps-0"> <div class="d-flex mb-2"> <div class="icon icon-shape icon-xxs shadow border-radius-sm bg-gradient-info text-center me-2 d-flex align-items-center justify-content-center"> <svg width="10px" height="10px" viewBox="0 0 40 40" version="1.1" xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink"> <title>spaceship</title> <g id="Basic-Elements" stroke="none" stroke-width="1" fill="none" fill-rule="evenodd"> <g id="Rounded-Icons" transform="translate(-1720.000000, -592.000000)" fill="#FFFFFF" fill-rule="nonzero"> <g id="Icons-with-opacity" transform="translate(1716.000000, 291.000000)"> <g id="spaceship" transform="translate(4.000000, 301.000000)"> <path class="color-background" d="M39.3,0.706666667 C38.9660984,0.370464027 38.5048767,0.192278529 38.0316667,0.216666667 C14.6516667,1.43666667 6.015,22.2633333 5.93166667,22.4733333 C5.68236407,23.0926189 5.82664679,23.8009159 6.29833333,24.2733333 L15.7266667,33.7016667 C16.2013871,34.1756798 16.9140329,34.3188658 17.535,34.065 C17.7433333,33.98 38.4583333,25.2466667 39.7816667,1.97666667 C39.8087196,1.50414529 39.6335979,1.04240574 39.3,0.706666667 Z M25.69,19.0233333 C24.7367525,19.9768687 23.3029475,20.2622391 22.0572426,19.7463614 C20.8115377,19.2304837 19.9992882,18.0149658 19.9992882,16.6666667 C19.9992882,15.3183676 20.8115377,14.1028496 22.0572426,13.5869719 C23.3029475,13.0710943 24.7367525,13.3564646 25.69,14.31 C26.9912731,15.6116662 26.9912731,17.7216672 25.69,19.0233333 L25.69,19.0233333 Z" id="Shape"></path> <path class="color-background" d="M1.855,31.4066667 C3.05106558,30.2024182 4.79973884,29.7296005 6.43969145,30.1670277 C8.07964407,30.6044549 9.36054508,31.8853559 9.7979723,33.5253085 C10.2353995,35.1652612 9.76258177,36.9139344 8.55833333,38.11 C6.70666667,39.9616667 0,40 0,40 C0,40 0,33.2566667 1.855,31.4066667 Z" id="Path"></path> <path class="color-background" d="M17.2616667,3.90166667 C12.4943643,3.07192755 7.62174065,4.61673894 4.20333333,8.04166667 C3.31200265,8.94126033 2.53706177,9.94913142 1.89666667,11.0416667 C1.5109569,11.6966059 1.61721591,12.5295394 2.155,13.0666667 L5.47,16.3833333 C8.55036617,11.4946947 12.5559074,7.25476565 17.2616667,3.90166667 L17.2616667,3.90166667 Z" id="color-2" opacity="0.598539807"></path> <path class="color-background" d="M36.0983333,22.7383333 C36.9280725,27.5056357 35.3832611,32.3782594 31.9583333,35.7966667 C31.0587397,36.6879974 30.0508686,37.4629382 28.9583333,38.1033333 C28.3033941,38.4890431 27.4704606,38.3827841 26.9333333,37.845 L23.6166667,34.53 C28.5053053,31.4496338 32.7452344,27.4440926 36.0983333,22.7383333 L36.0983333,22.7383333 Z" id="color-3" opacity="0.598539807"></path> </g> </g> </g> </g> </svg> </div> <p class="text-xs mt-1 mb-0 font-weight-bold">Clicks</p> </div> <h4 class="font-weight-bolder">2m</h4> <div class="progress w-75"> <div class="progress-bar bg-dark w-90" role="progressbar" aria-valuenow="90" aria-valuemin="0" aria-valuemax="100"></div> </div> </div> <div class="col-3 py-3 ps-0"> <div class="d-flex mb-2"> <div class="icon icon-shape icon-xxs shadow border-radius-sm bg-gradient-warning text-center me-2 d-flex align-items-center justify-content-center"> <svg width="10px" height="10px" viewBox="0 0 43 36" version="1.1" xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink"> <title>credit-card</title> <g id="Basic-Elements" stroke="none" stroke-width="1" fill="none" fill-rule="evenodd"> <g id="Rounded-Icons" transform="translate(-2169.000000, -745.000000)" fill="#FFFFFF" fill-rule="nonzero"> <g id="Icons-with-opacity" transform="translate(1716.000000, 291.000000)"> <g id="credit-card" transform="translate(453.000000, 454.000000)"> <path class="color-background" d="M43,10.7482083 L43,3.58333333 C43,1.60354167 41.3964583,0 39.4166667,0 L3.58333333,0 C1.60354167,0 0,1.60354167 0,3.58333333 L0,10.7482083 L43,10.7482083 Z" id="Path" opacity="0.593633743"></path> <path class="color-background" d="M0,16.125 L0,32.25 C0,34.2297917 1.60354167,35.8333333 3.58333333,35.8333333 L39.4166667,35.8333333 C41.3964583,35.8333333 43,34.2297917 43,32.25 L43,16.125 L0,16.125 Z M19.7083333,26.875 L7.16666667,26.875 L7.16666667,23.2916667 L19.7083333,23.2916667 L19.7083333,26.875 Z M35.8333333,26.875 L28.6666667,26.875 L28.6666667,23.2916667 L35.8333333,23.2916667 L35.8333333,26.875 Z" id="Shape"></path> </g> </g> </g> </g> </svg> </div> <p class="text-xs mt-1 mb-0 font-weight-bold">Sales</p> </div> <h4 class="font-weight-bolder">435$</h4> <div class="progress w-75"> <div class="progress-bar bg-dark w-30" role="progressbar" aria-valuenow="30" aria-valuemin="0" aria-valuemax="100"></div> </div> </div> <div class="col-3 py-3 ps-0"> <div class="d-flex mb-2"> <div class="icon icon-shape icon-xxs shadow border-radius-sm bg-gradient-danger text-center me-2 d-flex align-items-center justify-content-center"> <svg width="10px" height="10px" viewBox="0 0 40 40" version="1.1" xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink"> <title>settings</title> <g id="Basic-Elements" stroke="none" stroke-width="1" fill="none" fill-rule="evenodd"> <g id="Rounded-Icons" transform="translate(-2020.000000, -442.000000)" fill="#FFFFFF" fill-rule="nonzero"> <g id="Icons-with-opacity" transform="translate(1716.000000, 291.000000)"> <g id="settings" transform="translate(304.000000, 151.000000)"> <polygon class="color-background" id="Path" opacity="0.596981957" points="18.0883333 15.7316667 11.1783333 8.82166667 13.3333333 6.66666667 6.66666667 0 0 6.66666667 6.66666667 13.3333333 8.82166667 11.1783333 15.315 17.6716667"></polygon> <path class="color-background" d="M31.5666667,23.2333333 C31.0516667,23.2933333 30.53,23.3333333 30,23.3333333 C29.4916667,23.3333333 28.9866667,23.3033333 28.48,23.245 L22.4116667,30.7433333 L29.9416667,38.2733333 C32.2433333,40.575 35.9733333,40.575 38.275,38.2733333 L38.275,38.2733333 C40.5766667,35.9716667 40.5766667,32.2416667 38.275,29.94 L31.5666667,23.2333333 Z" id="Path" opacity="0.596981957"></path> <path class="color-background" d="M33.785,11.285 L28.715,6.215 L34.0616667,0.868333333 C32.82,0.315 31.4483333,0 30,0 C24.4766667,0 20,4.47666667 20,10 C20,10.99 20.1483333,11.9433333 20.4166667,12.8466667 L2.435,27.3966667 C0.95,28.7083333 0.0633333333,30.595 0.00333333333,32.5733333 C-0.0583333333,34.5533333 0.71,36.4916667 2.11,37.89 C3.47,39.2516667 5.27833333,40 7.20166667,40 C9.26666667,40 11.2366667,39.1133333 12.6033333,37.565 L27.1533333,19.5833333 C28.0566667,19.8516667 29.01,20 30,20 C35.5233333,20 40,15.5233333 40,10 C40,8.55166667 39.685,7.18 39.1316667,5.93666667 L33.785,11.285 Z" id="Path"></path> </g> </g> </g> </g> </svg> </div> <p class="text-xs mt-1 mb-0 font-weight-bold">Items</p> </div> <h4 class="font-weight-bolder">43</h4> <div class="progress w-75"> <div class="progress-bar bg-dark w-50" role="progressbar" aria-valuenow="50" aria-valuemin="0" aria-valuemax="100"></div> </div> </div> </div> </div> </div> </div>
<script>
var chartsBar = document.querySelectorAll(".chart-bar"); chartsBar.forEach(function(chart) { if (!chart.getAttribute('data-chart-initialized')) { new Chart(chart, { type: "bar", data: { labels: ["Apr", "May", "Jun", "Jul", "Aug", "Sep", "Oct", "Nov", "Dec"], datasets: [{ label: "Sales", tension: 0.4, borderWidth: 0, borderRadius: 4, borderSkipped: false, backgroundColor: "#fff", data: [450, 200, 100, 220, 500, 100, 400, 230, 500], maxBarThickness: 6, }, ], }, options: { responsive: true, maintainAspectRatio: false, plugins: { legend: { display: false, }, }, interaction: { intersect: false, mode: "index", }, scales: { y: { grid: { drawBorder: false, display: false, drawOnChartArea: false, drawTicks: false, }, ticks: { suggestedMin: 0, suggestedMax: 600, beginAtZero: true, padding: 15, font: { size: 14, family: "Open Sans", style: "normal", lineHeight: 2, }, color: "#fff", }, }, x: { grid: { drawBorder: false, display: false, drawOnChartArea: false, drawTicks: false, }, ticks: { display: false, }, }, }, }, }); chart.setAttribute("data-chart-initialized", "true"); } });
</script>
Requires JavaScript
Chart Pie
Component from
Asteria Dashboard
Builder
<div class="card mb-4 z-index-2 draggable"> <div class="card-header pb-0"> <h6 class="mb-1">Market Distribution</h6> </div> <div class="card-body card-body px-3 pt-lg-6 pb-lg-5"> <div class="row h-100"> <div class="col-lg-5 my-auto text-center d-lg-block d-flex justify-content-center"> <div id="chart-pie" class="chart-pie"> </div> </div> </div> </div> </div>
<script>
var ctx = document.getElementById("chart-pie"); var chartPie = new ApexCharts(ctx, { chart: { width: 380, type: 'donut', }, dataLabels: { enabled: false }, plotOptions: { pie: { customScale: 1, expandOnClick: false, donut: { size: "80%", } }, }, legend: { position: "right", verticalAlign: "center", containerMargin: { left: 35, right: 60 } }, series: [66, 55, 13, 33], labels: ['Asia', 'USA', 'China', 'Africa'], colors: ['#00ab5599', '#00ab55', '#00ab5535', '#00ab5550'], donut: { size: "100%" }, responsive: [ { breakpoint: 1550, options: { chart: { width: 340, }, legend: { position: "bottom", verticalAlign: "bottom", containerMargin: { left: 'auto', right: 'auto' } }, } }, { breakpoint: 1450, options: { chart: { width: 300, }, } } ] }); chartPie.render();
</script>
Requires JavaScript
Chart Lines
Component from
Asteria Dashboard
Builder
<div class="card mb-4 draggable"> <div class="card-header pb-0 d-flex align-items-center"> <div> <h6 class="mb-1">Sales overview</h6> <p class="text-sm mb-0"> (+32%) more in 2021 </p> </div> <select class="form-select form-select-sm ms-auto w-20 font-weight-bolder bg-gray-100" aria-label=".form-select-sm example"> <option selected>2021</option> <option value="2020">2020</option> </select> </div> <div class="card-body p-3"> <div class="chart"> <canvas class="chart-line" class="chart-canvas" height="300"></canvas> </div> </div> </div>
<script>
var ctx2 = document.querySelectorAll(".chart-line"); new Chart(ctx2[ctx2.length-1], { type: "line", data: { labels: ["Apr", "May", "Jun", "Jul", "Aug", "Sep", "Oct", "Nov", "Dec"], datasets: [{ label: "Black Friday", tension: 0.4, borderWidth: 0, pointRadius: 0, borderColor: "#00ab55", borderWidth: 3, backgroundColor: "transparent", data: [20, 60, 20, 50, 90, 220, 440, 380, 500], maxBarThickness: 6 }, { label: "Autumn Sale", tension: 0.4, borderWidth: 0, pointRadius: 0, borderColor: "#212b36", borderWidth: 3, backgroundColor: "transparent", data: [30, 90, 40, 140, 290, 290, 240, 270, 230], maxBarThickness: 6 }, ], }, options: { responsive: true, maintainAspectRatio: false, legend: { display: false, }, tooltips: { enabled: true, mode: "index", intersect: false, }, scales: { yAxes: [{ gridLines: { borderDash: [2], borderDashOffset: [2], color: '#dee2e6', zeroLineColor: '#dee2e6', zeroLineWidth: 1, zeroLineBorderDash: [2], drawBorder: false, }, ticks: { suggestedMin: 0, suggestedMax: 500, beginAtZero: true, padding: 10, fontSize: 11, fontColor: '#adb5bd', lineHeight: 3, fontStyle: 'normal', fontFamily: "Public Sans", }, }, ], xAxes: [{ gridLines: { zeroLineColor: 'rgba(0,0,0,0)', display: false, }, ticks: { padding: 10, fontSize: 11, fontColor: '#adb5bd', lineHeight: 3, fontStyle: 'normal', fontFamily: "Public Sans", }, }, ], }, }, });
</script>
Requires JavaScript
Chart Line Nav
Component from
Argon Dashboard
Builder
<div class="card bg-default draggable"> <div class="card-header bg-transparent"> <div class="row align-items-center"> <div class="col"> <h6 class="text-light text-uppercase ls-1 mb-1">Overview</h6> <h5 class="h3 text-white mb-0">Sales value</h5> </div> <div class="col"> <ul class="nav nav-pills justify-content-end"> <li class="nav-item mr-2 mr-md-0" data-toggle="chart"> <a href="#" class="nav-link py-2 px-3 active" data-toggle="tab"> <span class="d-none d-md-block">Month</span> <span class="d-md-none">M</span> </a> </li> <li class="nav-item" data-toggle="chart"> <a href="#" class="nav-link py-2 px-3" data-toggle="tab"> <span class="d-none d-md-block">Week</span> <span class="d-md-none">W</span> </a> </li> </ul> </div> </div> </div> <div class="card-body"> <div class="chart"> <canvas id="chart-sales-dark" class="chart-line chart-canvas"></canvas> </div> </div> </div>
<script>
var chartsLine = document.querySelectorAll(".chart-line"); chartsLine.forEach(function(chart) { if (!chart.getAttribute('data-chart-initialized')) { new Chart(chart, { type: "line", data: { labels: ["May", "Jun", "Jul", "Aug", "Sep", "Oct", "Nov", "Dec"], datasets: [{ label: "Performance", tension: 0.4, borderWidth: 4, borderColor: "#5e72e4", pointRadius: 0, backgroundColor: "transparent", data: [0, 20, 10, 30, 15, 40, 20, 60, 60], }, ], }, options: { responsive: true, maintainAspectRatio: false, legend: { display: false, }, tooltips: { enabled: true, mode: "index", intersect: false, }, scales: { yAxes: [{ barPercentage: 1.6, gridLines: { drawBorder: false, color: "rgba(29,140,248,0.0)", zeroLineColor: "transparent", }, ticks: { padding: 0, fontColor: "#8898aa", fontSize: 13, fontFamily: "Open Sans", }, }, ], xAxes: [{ barPercentage: 1.6, gridLines: { drawBorder: false, color: "rgba(29,140,248,0.0)", zeroLineColor: "transparent", }, ticks: { padding: 10, fontColor: "#8898aa", fontSize: 13, fontFamily: "Open Sans", }, }, ], }, layout: { padding: 0, }, }, }); chart.setAttribute("data-chart-initialized", "true"); } });
</script>
Requires JavaScript
Chart Line
Component from
Adminkit
Builder
<div class="card flex-fill w-100 draggable"> <div class="card-header"> <h5 class="card-title mb-0">Recent Movement</h5> </div> <div class="card-body py-3"> <div class="chart chart-sm"><div class="chartjs-size-monitor"><div class="chartjs-size-monitor-expand"><div class=""></div></div><div class="chartjs-size-monitor-shrink"><div class=""></div></div></div> <canvas id="chartjs-dashboard-line" style="display: block; height: 252px; width: 428px;" width="856" height="504" class="chart-line chartjs-render-monitor"></canvas> </div> </div> </div>
<script>
var chartsLine = document.querySelectorAll(".chart-line"); chartsLine.forEach(function(chart) { if (!chart.getAttribute('data-chart-initialized')) { var ctx = chart.getContext("2d"); var gradient = ctx.createLinearGradient(0, 0, 0, 225); gradient.addColorStop(0, "rgba(215, 227, 244, 1)"); gradient.addColorStop(1, "rgba(215, 227, 244, 0)"); // Line chart new Chart(ctx, { type: "line", data: { labels: ["Jan", "Feb", "Mar", "Apr", "May", "Jun", "Jul", "Aug", "Sep", "Oct", "Nov", "Dec"], datasets: [{ label: "Sales ($)", fill: true, backgroundColor: gradient, borderColor: window.theme.primary, data: [ 2115, 1562, 1584, 1892, 1587, 1923, 2566, 2448, 2805, 3438, 2917, 3327 ] }] }, options: { maintainAspectRatio: false, legend: { display: false }, tooltips: { intersect: false }, hover: { intersect: true }, plugins: { filler: { propagate: false } }, scales: { xAxes: [{ reverse: true, gridLines: { color: "rgba(0,0,0,0.0)" } }], yAxes: [{ ticks: { stepSize: 1000 }, display: true, borderDash: [3, 3], gridLines: { color: "rgba(0,0,0,0.0)" } }] } } }); chart.setAttribute("data-chart-initialized", "true"); } });
</script>
Requires JavaScript
Chart Pie
Component from
Adminkit
Builder
<div class="card flex-fill w-100 draggable"> <div class="card-header"> <h5 class="card-title mb-0">Browser Usage</h5> </div> <div class="card-body d-flex"> <div class="align-self-center w-100"> <div class="py-3"> <div class="chart chart-xs"><div class="chartjs-size-monitor"><div class="chartjs-size-monitor-expand"><div class=""></div></div><div class="chartjs-size-monitor-shrink"><div class=""></div></div></div> <canvas id="chartjs-dashboard-pie" width="856" height="400" style="display: block; height: 200px; width: 428px;" class="chart-pie chartjs-render-monitor"></canvas> </div> </div> <table class="table mb-0"> <tbody> <tr> <td>Chrome</td> <td class="text-end">4306</td> </tr> <tr> <td>Firefox</td> <td class="text-end">3801</td> </tr> <tr> <td>IE</td> <td class="text-end">1689</td> </tr> </tbody> </table> </div> </div> </div>
<script>
var chartsPie = document.querySelectorAll(".chart-pie"); chartsPie.forEach(function(chart) { if (!chart.getAttribute('data-chart-initialized')) { new Chart(chart, { type: "pie", data: { labels: ["Chrome", "Firefox", "IE"], datasets: [{ data: [4306, 3801, 1689], backgroundColor: [ window.theme.primary, window.theme.warning, window.theme.danger ], borderWidth: 5 }] }, options: { responsive: !window.MSInputMethodContext, maintainAspectRatio: false, legend: { display: false }, cutoutPercentage: 75 } }); chart.setAttribute("data-chart-initialized", "true"); } });
</script>
Requires JavaScript
Chart Bar
Component from
Soft UI Dashboard Tailwind
Builder
<div class="border-black/12.5 shadow-soft-xl relative z-20 flex min-w-0 flex-col break-words rounded-2xl border-0 border-solid bg-white bg-clip-border mb-4 draggable"> <div class="flex-auto p-4"> <div class="py-4 pr-1 mb-4 bg-gradient-to-tl from-gray-900 to-slate-800 rounded-xl"> <div> <canvas id="chart-bars" class="chart-canvas chart-bars" height="340" width="655" style="display: block; box-sizing: border-box; height: 170px; width: 327.5px;"></canvas> </div> </div> <h6 class="mt-6 mb-0 ml-2 font-display">Active Users</h6> <p class="ml-2 leading-normal text-sm">( <span class="font-bold">+23%</span>) than last week </p> <div class="w-full px-6 mx-auto max-w-screen-2xl rounded-xl"> <div class="flex flex-wrap mt-0 -mx-3"> <div class="flex-none w-1/4 max-w-full py-4 pl-0 pr-3 mt-0"> <div class="flex mb-2"> <div class="flex items-center justify-center w-5 h-5 mr-2 text-center bg-center rounded fill-current shadow-soft-2xl bg-gradient-to-tl from-purple-700 to-pink-500 text-neutral-900"> <svg width="10px" height="10px" viewBox="0 0 40 44" version="1.1" xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink"> <title>document</title> <g stroke="none" stroke-width="1" fill="none" fill-rule="evenodd"> <g transform="translate(-1870.000000, -591.000000)" fill="#FFFFFF" fill-rule="nonzero"> <g transform="translate(1716.000000, 291.000000)"> <g transform="translate(154.000000, 300.000000)"> <path class="color-background" d="M40,40 L36.3636364,40 L36.3636364,3.63636364 L5.45454545,3.63636364 L5.45454545,0 L38.1818182,0 C39.1854545,0 40,0.814545455 40,1.81818182 L40,40 Z" opacity="0.603585379"></path> <path class="color-background" d="M30.9090909,7.27272727 L1.81818182,7.27272727 C0.814545455,7.27272727 0,8.08727273 0,9.09090909 L0,41.8181818 C0,42.8218182 0.814545455,43.6363636 1.81818182,43.6363636 L30.9090909,43.6363636 C31.9127273,43.6363636 32.7272727,42.8218182 32.7272727,41.8181818 L32.7272727,9.09090909 C32.7272727,8.08727273 31.9127273,7.27272727 30.9090909,7.27272727 Z M18.1818182,34.5454545 L7.27272727,34.5454545 L7.27272727,30.9090909 L18.1818182,30.9090909 L18.1818182,34.5454545 Z M25.4545455,27.2727273 L7.27272727,27.2727273 L7.27272727,23.6363636 L25.4545455,23.6363636 L25.4545455,27.2727273 Z M25.4545455,20 L7.27272727,20 L7.27272727,16.3636364 L25.4545455,16.3636364 L25.4545455,20 Z"></path> </g> </g> </g> </g> </svg> </div> <p class="mt-1 mb-0 font-semibold leading-tight text-xs">Users</p> </div> <h4 class="font-bold font-display">36K</h4> <div class="text-xs h-0.75 flex w-3/4 overflow-visible rounded-lg bg-gray-200"> <div class="duration-600 ease-soft -mt-0.38 -ml-px flex h-1.5 w-3/5 flex-col justify-center overflow-hidden whitespace-nowrap rounded-lg bg-slate-700 text-center text-white transition-all" role="progressbar" aria-valuenow="60" aria-valuemin="0" aria-valuemax="100"></div> </div> </div> <div class="flex-none w-1/4 max-w-full py-4 pl-0 pr-3 mt-0"> <div class="flex mb-2"> <div class="flex items-center justify-center w-5 h-5 mr-2 text-center bg-center rounded fill-current shadow-soft-2xl bg-gradient-to-tl from-blue-600 to-cyan-400 text-neutral-900"> <svg width="10px" height="10px" viewBox="0 0 40 40" version="1.1" xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink"> <title>spaceship</title> <g stroke="none" stroke-width="1" fill="none" fill-rule="evenodd"> <g transform="translate(-1720.000000, -592.000000)" fill="#FFFFFF" fill-rule="nonzero"> <g transform="translate(1716.000000, 291.000000)"> <g transform="translate(4.000000, 301.000000)"> <path class="color-background" d="M39.3,0.706666667 C38.9660984,0.370464027 38.5048767,0.192278529 38.0316667,0.216666667 C14.6516667,1.43666667 6.015,22.2633333 5.93166667,22.4733333 C5.68236407,23.0926189 5.82664679,23.8009159 6.29833333,24.2733333 L15.7266667,33.7016667 C16.2013871,34.1756798 16.9140329,34.3188658 17.535,34.065 C17.7433333,33.98 38.4583333,25.2466667 39.7816667,1.97666667 C39.8087196,1.50414529 39.6335979,1.04240574 39.3,0.706666667 Z M25.69,19.0233333 C24.7367525,19.9768687 23.3029475,20.2622391 22.0572426,19.7463614 C20.8115377,19.2304837 19.9992882,18.0149658 19.9992882,16.6666667 C19.9992882,15.3183676 20.8115377,14.1028496 22.0572426,13.5869719 C23.3029475,13.0710943 24.7367525,13.3564646 25.69,14.31 C26.9912731,15.6116662 26.9912731,17.7216672 25.69,19.0233333 L25.69,19.0233333 Z"></path> <path class="color-background" d="M1.855,31.4066667 C3.05106558,30.2024182 4.79973884,29.7296005 6.43969145,30.1670277 C8.07964407,30.6044549 9.36054508,31.8853559 9.7979723,33.5253085 C10.2353995,35.1652612 9.76258177,36.9139344 8.55833333,38.11 C6.70666667,39.9616667 0,40 0,40 C0,40 0,33.2566667 1.855,31.4066667 Z"></path> <path class="color-background" d="M17.2616667,3.90166667 C12.4943643,3.07192755 7.62174065,4.61673894 4.20333333,8.04166667 C3.31200265,8.94126033 2.53706177,9.94913142 1.89666667,11.0416667 C1.5109569,11.6966059 1.61721591,12.5295394 2.155,13.0666667 L5.47,16.3833333 C8.55036617,11.4946947 12.5559074,7.25476565 17.2616667,3.90166667 L17.2616667,3.90166667 Z" opacity="0.598539807"></path> <path class="color-background" d="M36.0983333,22.7383333 C36.9280725,27.5056357 35.3832611,32.3782594 31.9583333,35.7966667 C31.0587397,36.6879974 30.0508686,37.4629382 28.9583333,38.1033333 C28.3033941,38.4890431 27.4704606,38.3827841 26.9333333,37.845 L23.6166667,34.53 C28.5053053,31.4496338 32.7452344,27.4440926 36.0983333,22.7383333 L36.0983333,22.7383333 Z" opacity="0.598539807"></path> </g> </g> </g> </g> </svg> </div> <p class="mt-1 mb-0 font-semibold leading-tight text-xs">Clicks</p> </div> <h4 class="font-bold font-display">2m</h4> <div class="text-xs h-0.75 flex w-3/4 overflow-visible rounded-lg bg-gray-200"> <div class="duration-600 ease-soft -mt-0.38 w-9/10 -ml-px flex h-1.5 flex-col justify-center overflow-hidden whitespace-nowrap rounded-lg bg-slate-700 text-center text-white transition-all" role="progressbar" aria-valuenow="90" aria-valuemin="0" aria-valuemax="100"></div> </div> </div> <div class="flex-none w-1/4 max-w-full py-4 pl-0 pr-3 mt-0"> <div class="flex mb-2"> <div class="flex items-center justify-center w-5 h-5 mr-2 text-center bg-center rounded fill-current shadow-soft-2xl bg-gradient-to-tl from-red-500 to-yellow-400 text-neutral-900"> <svg width="10px" height="10px" viewBox="0 0 43 36" version="1.1" xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink"> <title>credit-card</title> <g stroke="none" stroke-width="1" fill="none" fill-rule="evenodd"> <g transform="translate(-2169.000000, -745.000000)" fill="#FFFFFF" fill-rule="nonzero"> <g transform="translate(1716.000000, 291.000000)"> <g transform="translate(453.000000, 454.000000)"> <path class="color-background" d="M43,10.7482083 L43,3.58333333 C43,1.60354167 41.3964583,0 39.4166667,0 L3.58333333,0 C1.60354167,0 0,1.60354167 0,3.58333333 L0,10.7482083 L43,10.7482083 Z" opacity="0.593633743"></path> <path class="color-background" d="M0,16.125 L0,32.25 C0,34.2297917 1.60354167,35.8333333 3.58333333,35.8333333 L39.4166667,35.8333333 C41.3964583,35.8333333 43,34.2297917 43,32.25 L43,16.125 L0,16.125 Z M19.7083333,26.875 L7.16666667,26.875 L7.16666667,23.2916667 L19.7083333,23.2916667 L19.7083333,26.875 Z M35.8333333,26.875 L28.6666667,26.875 L28.6666667,23.2916667 L35.8333333,23.2916667 L35.8333333,26.875 Z"></path> </g> </g> </g> </g> </svg> </div> <p class="mt-1 mb-0 font-semibold leading-tight text-xs">Sales</p> </div> <h4 class="font-bold font-display">435$</h4> <div class="text-xs h-0.75 flex w-3/4 overflow-visible rounded-lg bg-gray-200"> <div class="duration-600 ease-soft -mt-0.38 w-3/10 -ml-px flex h-1.5 flex-col justify-center overflow-hidden whitespace-nowrap rounded-lg bg-slate-700 text-center text-white transition-all" role="progressbar" aria-valuenow="30" aria-valuemin="0" aria-valuemax="100"></div> </div> </div> <div class="flex-none w-1/4 max-w-full py-4 pl-0 pr-3 mt-0"> <div class="flex mb-2"> <div class="flex items-center justify-center w-5 h-5 mr-2 text-center bg-center rounded fill-current shadow-soft-2xl bg-gradient-to-tl from-red-600 to-rose-400 text-neutral-900"> <svg width="10px" height="10px" viewBox="0 0 40 40" version="1.1" xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink"> <title>settings</title> <g stroke="none" stroke-width="1" fill="none" fill-rule="evenodd"> <g transform="translate(-2020.000000, -442.000000)" fill="#FFFFFF" fill-rule="nonzero"> <g transform="translate(1716.000000, 291.000000)"> <g transform="translate(304.000000, 151.000000)"> <polygon class="color-background" opacity="0.596981957" points="18.0883333 15.7316667 11.1783333 8.82166667 13.3333333 6.66666667 6.66666667 0 0 6.66666667 6.66666667 13.3333333 8.82166667 11.1783333 15.315 17.6716667"></polygon> <path class="color-background" d="M31.5666667,23.2333333 C31.0516667,23.2933333 30.53,23.3333333 30,23.3333333 C29.4916667,23.3333333 28.9866667,23.3033333 28.48,23.245 L22.4116667,30.7433333 L29.9416667,38.2733333 C32.2433333,40.575 35.9733333,40.575 38.275,38.2733333 L38.275,38.2733333 C40.5766667,35.9716667 40.5766667,32.2416667 38.275,29.94 L31.5666667,23.2333333 Z" opacity="0.596981957"></path> <path class="color-background" d="M33.785,11.285 L28.715,6.215 L34.0616667,0.868333333 C32.82,0.315 31.4483333,0 30,0 C24.4766667,0 20,4.47666667 20,10 C20,10.99 20.1483333,11.9433333 20.4166667,12.8466667 L2.435,27.3966667 C0.95,28.7083333 0.0633333333,30.595 0.00333333333,32.5733333 C-0.0583333333,34.5533333 0.71,36.4916667 2.11,37.89 C3.47,39.2516667 5.27833333,40 7.20166667,40 C9.26666667,40 11.2366667,39.1133333 12.6033333,37.565 L27.1533333,19.5833333 C28.0566667,19.8516667 29.01,20 30,20 C35.5233333,20 40,15.5233333 40,10 C40,8.55166667 39.685,7.18 39.1316667,5.93666667 L33.785,11.285 Z"></path> </g> </g> </g> </g> </svg> </div> <p class="mt-1 mb-0 font-semibold leading-tight text-xs">Items</p> </div> <h4 class="font-bold font-display">43</h4> <div class="text-xs h-0.75 flex w-3/4 overflow-visible rounded-lg bg-gray-200"> <div class="duration-600 ease-soft -mt-0.38 -ml-px flex h-1.5 w-1/2 flex-col justify-center overflow-hidden whitespace-nowrap rounded-lg bg-slate-700 text-center text-white transition-all" role="progressbar" aria-valuenow="50" aria-valuemin="0" aria-valuemax="100"></div> </div> </div> </div> </div> </div> </div>
<script>
var chartsBars = document.querySelectorAll(".chart-bars"); chartsBars.forEach(function (chart) { if (!chart.getAttribute('data-chart-initialized')) { new Chart(chart, { type: "bar", data: { labels: ["Apr", "May", "Jun", "Jul", "Aug", "Sep", "Oct", "Nov", "Dec"], datasets: [{ label: "Sales", tension: 0.4, borderWidth: 0, borderRadius: 4, borderSkipped: false, backgroundColor: "#fff", data: [450, 200, 100, 220, 500, 100, 400, 230, 500], maxBarThickness: 6, }, ], }, options: { responsive: true, maintainAspectRatio: false, plugins: { legend: { display: false, }, }, interaction: { intersect: false, mode: "index", }, scales: { y: { grid: { drawBorder: false, display: false, drawOnChartArea: false, drawTicks: false, }, ticks: { suggestedMin: 0, suggestedMax: 600, beginAtZero: true, padding: 15, font: { size: 14, family: "Open Sans", style: "normal", lineHeight: 2, }, color: "#fff", }, }, x: { grid: { drawBorder: false, display: false, drawOnChartArea: false, drawTicks: false, }, ticks: { display: false, }, }, }, }, }); chart.setAttribute("data-chart-initialized", "true"); } });
</script>